JavaScript is a widespread programming language. Oddly enough, using a Web Browser like Chrome, Firefox or Brave is a great way to start learning JavaScript. Download one of these browsers and we can get started almost immediately after opening it up. For our examples we will be using Chrome, as it is currently the most widely used web browser.
Why JavaScript?
There is so much that you can do with JavaScript. You can make pasta fall from the sky, create a todo list, or go wherever your heart takes you. JavaScript is a key part of how you interact with websites. If you aren’t sold yet, try reading this post: Why should I learn JavaScript.
Setting Up
The first step is to download and set up a browser, if you are reading this post you have likely already done this. Hopefully you are using a browser mentioned at the start; Chrome. Create a new window and we will open up a hidden part of the browser; the console. The console can be accessed by going to Menu > More Tools > Developer Tools and clicking, or by using the keyboard shortcuts: Ctrl + Shift + J (Windows), Cmd + Option + J (Mac). Once you have the console open it should look something like this.
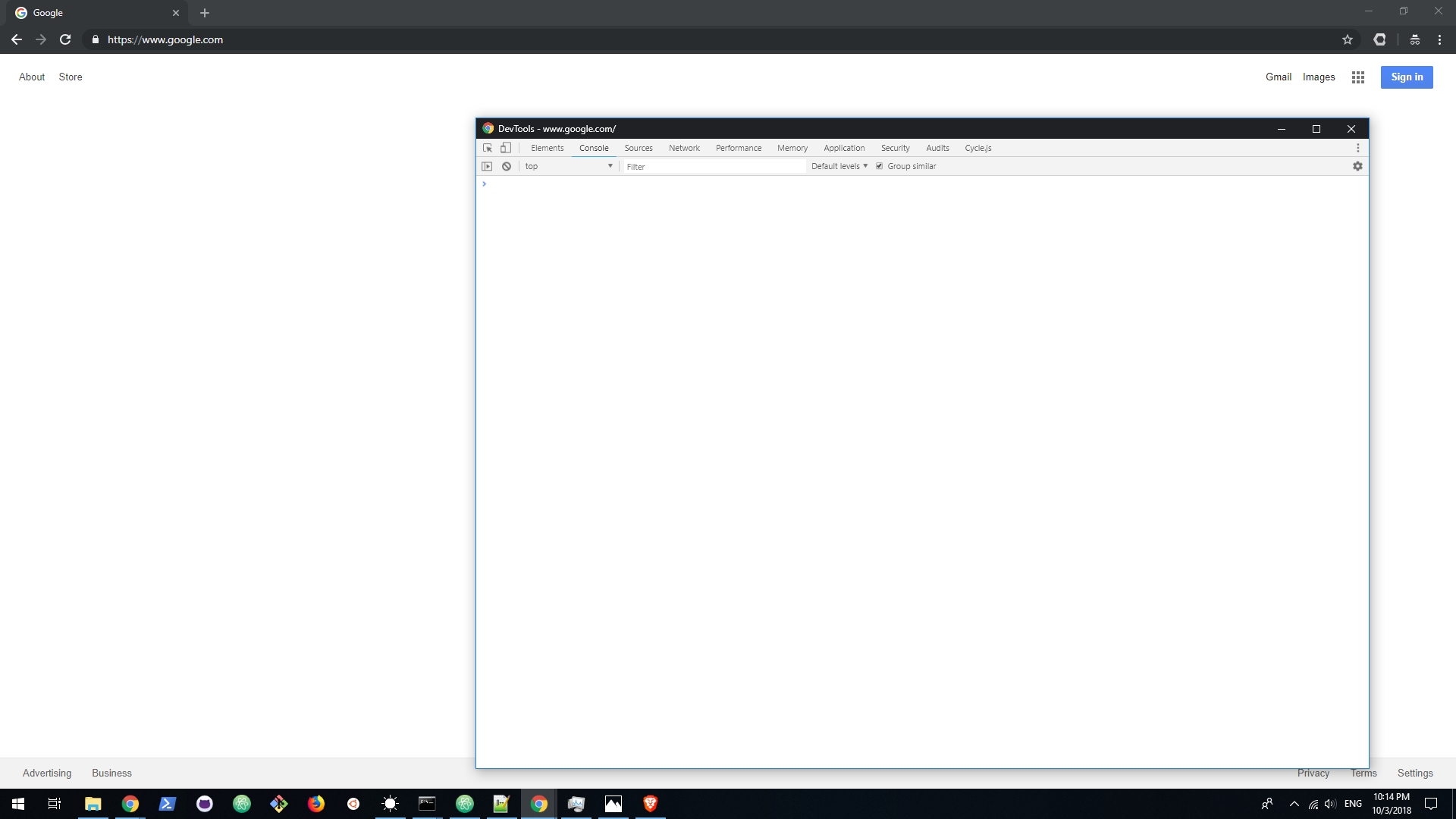
Using the console
Now we have the developer tools open and with the Console tab active. We can now start to use JavaScript to directly interact with our browser window! Type the following into the console and hit enter when you are done:
document.write('Hi!');
This will make windows content contain the text “Hi!”. Try changing the message. Type in the following code and hit enter at the console:
document.write(' I am learning and using JavaScript!');
Now the “Hi!” is followed by the new message. Pretty neat. We have used the console to interact with our browser window. Realistically you are not going to use document.write
very often, if at all. Now we are going to use the console to further explore the basics of JavaScript.
Working in the console
You might have noticed that after we typed our document.write commands we get an undefined message in the console below each command. When we type and execute commands in the console we get an output back out. When we run document.write, it does not return anything, so the console shows undefined. If we type 1 into the console and hit enter let’s see what the output value ends up as.
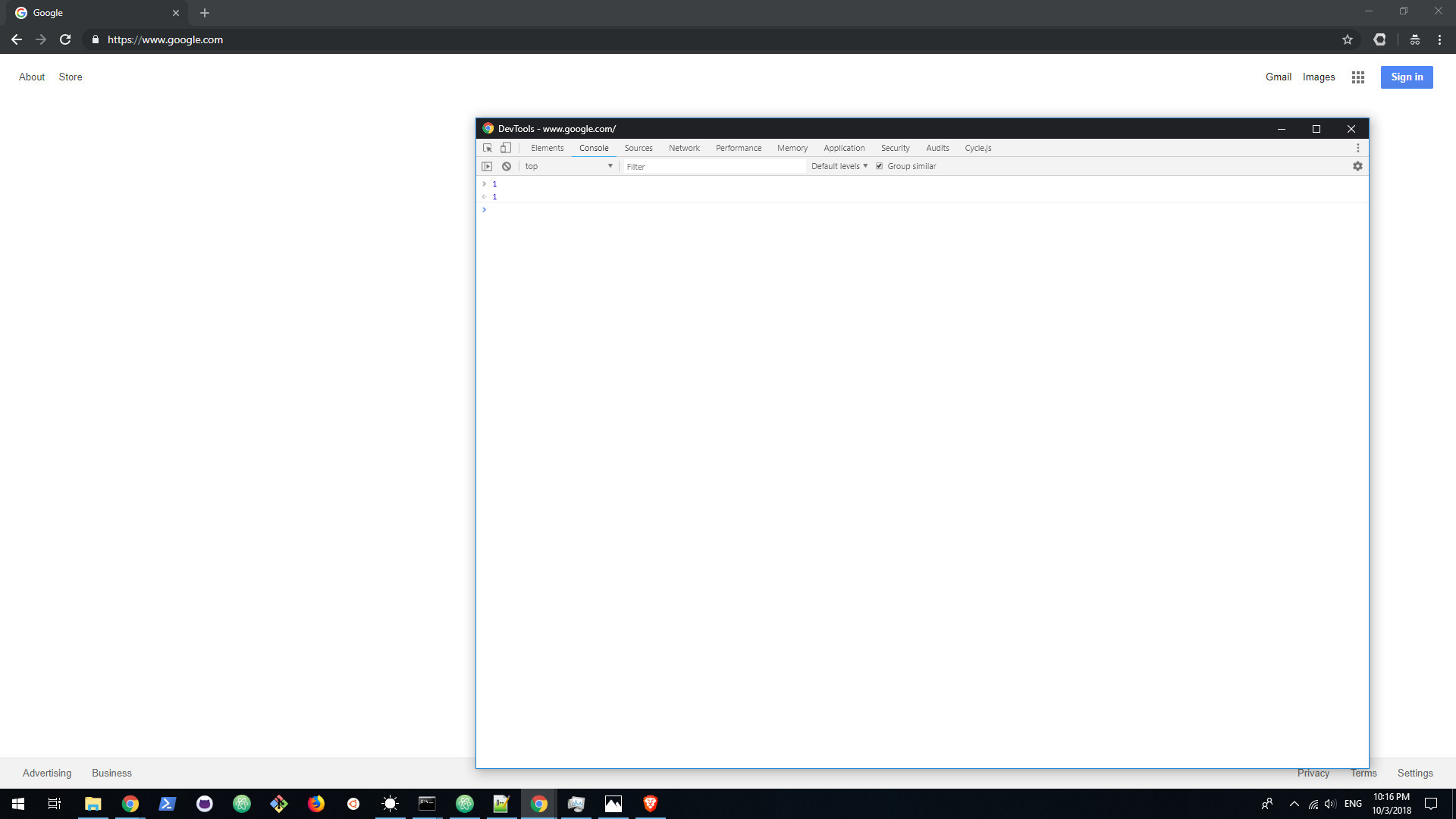
As expected we get 1
. Now we can do simple addition try:
11 + 11
We get an output of 22. There are some basic math operators we can use in JavaScript
- Addition: 2 + 2
- Outputs 4
- Subtraction: 3 – 2
- Outputs 1
- Multiplication: 3 * 2
- Outputs 6
- Division: 6 / 2
- Outputs 3
Working with decimals and other numbers can get a bit weird in JavaScript, so we will not go into too much depth for now. These numbers are a primitive data type in JavaScript, the other main types you will work with are strings, arrays, objects, and functions. Here is what each looks like in code.
- String
'Hi, I am a string!'
- Array
[ 1, 2, 3, ]
- Object
{ is: 'Object' }
- Function
function get10 () { return 10; }
Together these five primitives will be almost all you ever use in JavaScript. The challenge in JavaScript is figuring out how to arrange each of these pieces to create and explore your vision.